1. 문제
2. Book 객체를 활용하는 사례이다.
(1) 세 개의 == 연산자 함수를 가진 Book 클래스를 작성하라.
2. 결과
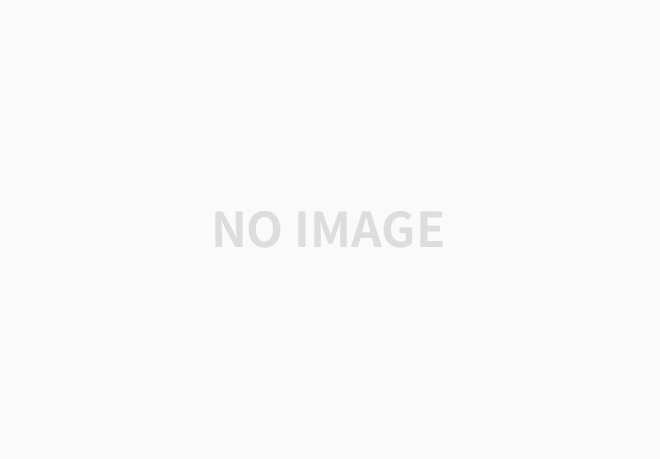
3. 코드
4. 설명
연산자 "=="를 오버로딩하여서 매개 변수가 다른 함수 3개를 구현합니다.
매개변수가 int 형일 시 price와 비교를 하도록 구현하고, string 형 일시 title과 비교하도록합니다.
또한, Book 클래스와 사용했을 시 tilte, price, pages를 비교하여서 다 같다면 return true;를 아니면 return false;를 해줍니다.
1. 문제
2. Book 객체를 활용하는 사례이다.
(2). 세 개의 == 연산자를 프렌드 함수로 작성하라.
2. 결과
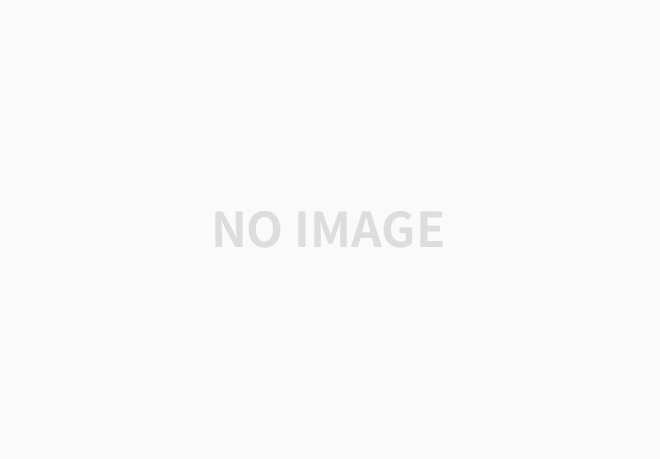
3. 코드
4. 설명
(1)번과 같은 기능을 하는 == 연산자 기능을 하는 함수들을 프렌드 함수로 작성하면 됩니다.
Book b 변수를 추가적으로 매개변수로 받아서 이용한다는 점과 friend를 붙이는 것 빼고는 구현하는 방법은 똑같습니다.
'명품 C++ programming' 카테고리의 다른 글
명품 C++ programming 실습문제 7장 5번 (0) | 2019.04.16 |
---|---|
명품 C++ programming 실습문제 7장 3번, 4번 (0) | 2019.04.16 |
명품 C++ programming 실습문제 7장 1번 (0) | 2019.04.15 |
명품 C++ programming 6장 Open Challenge (0) | 2019.04.14 |
명품 C++ programming 실습문제 6장 8번 (0) | 2019.04.13 |